Updated May 12, 2025 12 min read
Regression vs Unit Testing: A Comprehensive Guide
Unit and regression testing may seem similar, but they serve different purposes at different stages of the development process.. In this article, we’ll break down their key differences and explain why mastering both is vital for delivering software users can trust.
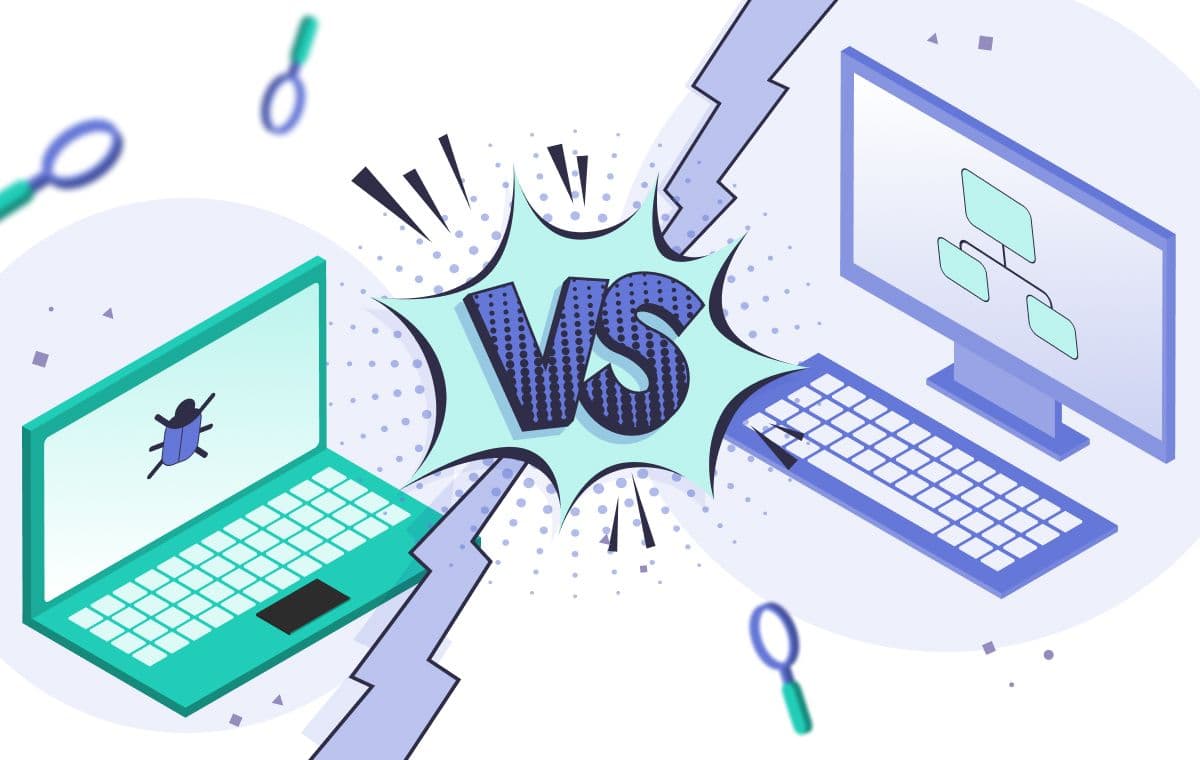
Despite their shared goal of ensuring software quality, unit testing and regression testing differ in their approaches. It's like testing the engine separately versus checking the entire plane before takeoff. The first ensures that the key components work correctly. The second ensures that all systems work together, and no change has disrupted their functionality. Both are critical if you want to "take off" without any issues.
According to industry data, over 80% of bugs found in production environments are caused by unexpected side effects of code changes. Unit tests can catch issues within a specific module early in development, but only regression testing ensures that changes in one module don't break functionality in other modules. Without it, critical bugs often go unnoticed until it’s too late.
In this article, we’ll break down the differences between unit testing and regression testing, explore their roles, show how they complement each other, and help you decide when and how to use each.
Unit Testing
Unit testing is a software testing method that involves testing each smallest possible testable component of a program in isolation. In procedural programming, including Java and C, a unit typically refers to a separate function, procedure, or program module. In object-oriented programming languages like Python and C++, it can refer to an interface, object, or class. Unit tests are typically written and maintained by developers as part of the development process to catch bugs early and ensure each component behaves as expected.
When testing relatively small modules—typically between 100 and 1,000 lines of code—it’s often possible to check many (if not all) logical branches of implementation, different paths in the data dependency graph, and boundary values of parameters. Based on this, various test coverage criteria are established, including full statement coverage, branch coverage, and boundary value coverage. (Discover how we help startups launch stable, well-tested products. Explore our software testing services for startups.)
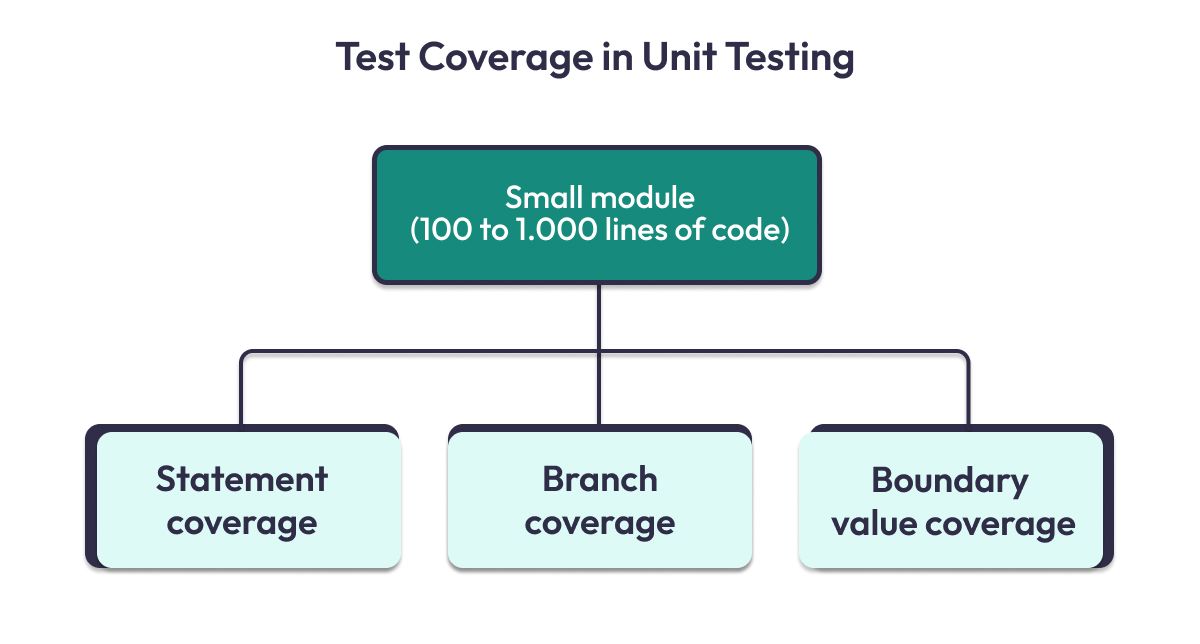
Unit testing is generally performed for each independent software module and is probably the most common form of testing, especially in small and medium-sized systems.
It’s important to note that software testing cannot uncover every bug in a program. In most software, it's practically impossible to test every execution path. The same holds true for unit testing—it can confirm the presence of bugs, but not their absence. Moreover, unit testing focuses solely on individual units and is not capable of identifying integration issues or other broader problems, such as architectural flaws or performance under load.
One of the most effective approaches to unit (component) testing is to prepare automated tests before writing the actual code, as practised in extreme programming. This is known as test-driven development (TDD) or the test-first approach. In this approach, small portions of code are written and integrated, and corresponding tests, written beforehand, are executed to validate their functionality. Development continues until all tests pass successfully.
Goals and Objectives of Unit Testing
The main goal of unit testing is to verify that each individual component (method, class, module) meets its specified requirements before it is integrated into the system. In systems developed using procedural languages, a test driver is created for each module to invoke its functions and collect the results. Stubs are used to simulate the behaviour of other modules that are not being tested at that moment. In object-oriented systems, unit testing has its specific features, primarily due to the encapsulation of data and methods within classes.
- Drivers are test modules that execute the component under test.
- Stubs replace the missing components that are invoked by the component under test and may:
- Return predetermined values immediately without performing any logic
- Display trace messages and allow the tester to proceed manually
- Return constant values or request input from the tester
- Provide a simplified implementation of the missing component
- Simulate exceptional or error conditions.
By evaluating each component in isolation and confirming its correctness, defects can be identified much earlier than if the component were already integrated into the system.
Unit testing is most effective in identifying defects related to:
- Algorithmic and coding errors
- Loop and conditional logic
- Use of local variables and resources.
However, defects related to data misinterpretation, incorrect interface implementation, compatibility, performance, etc., are typically not detected at the unit level and are discovered in higher levels of testing. You can also check out our article on how to do unit testing to explore the basics, tools, and best practices.
Unit Testing Strategy and Challenges
The strategy for unit testing—i.e., how test inputs are selected—depends on the effectiveness in uncovering specific types of bugs. Software development organisations often maintain a repository of historical project data, including build versions, design decisions, errors, and successes. By analysing previous versions, developers can avoid recurring mistakes, for example, identifying which types of defects are best caught at which testing stage.
In this case, we analyse the unit testing stage. If the analysis doesn’t yield useful insights (e.g., if the corresponding modules were not built in previous versions), the primary focus shifts to identifying local defects, where the code, resources, and data involved are specific to the module being tested.
For example, at the unit level, errors in parameter handling algorithms can be caught relatively easily, while issues related to the order or format of parameters (which involve other modules or interface specifications) might be missed.
Because the modules tested are generally small in size, unit testing is considered the simplest (though still labour-intensive) stage of testing. However, despite its apparent simplicity, unit testing presents two main challenges:
- Lack of a universal definition of what exactly constitutes a "module."
- Different interpretations of unit testing itself—whether it means testing the module in isolation within a test environment, or checking its behaviour as part of a functioning system.
Regression Testing
A fundamental challenge in software maintenance is that fixing one bug is highly likely (20–50%) to introduce a new one. As a result, the process often follows a “two steps forward, one step back” pattern. Because of this, maintaining software typically requires significantly more systematic debugging per line of code. Theoretically, after any changes in code (whether it’s an update, a bug fix, or a new feature), the entire set of test cases previously used to verify the system should be re-executed to ensure that nothing has been unintentionally broken. In practice, such regression testing should ideally come as close as possible to this theoretical model, although it is often costly.
Regression testing is a general term for all types of software testing, performed by QA engineers, aimed at detecting bugs in previously tested areas of the codebase. These bugs, where something that used to work correctly fails after changes are introduced, are called regression bugs.
One of the main goals of regression testing is to determine whether changes made in one part of the software impact other parts of the system. A good practice when QA finds a bug (if the test case didn’t exist before) is to create a test case that reproduces the issue and run it regularly after future changes. Regression testing can be performed manually or using specialised tools for automated execution. In some projects, tools are used to automatically run regression tests at regular intervals, either after changes to the existing build or after creating a new build.
Goals, Objectives of Regression Testing
Over time, the system changes, either due to bug fixes or enhancements in functionality. Software testing is not a one-time event but a continuous process throughout the software development lifecycle. The role of the tester in such scenarios is to confirm that the new or updated functionality has not introduced new bugs, and if issues do arise, to identify their causes. The simplest and most effective method is to re-execute the complete test suite after every significant system change. In general, re-executing the tests can result in one of three outcomes:
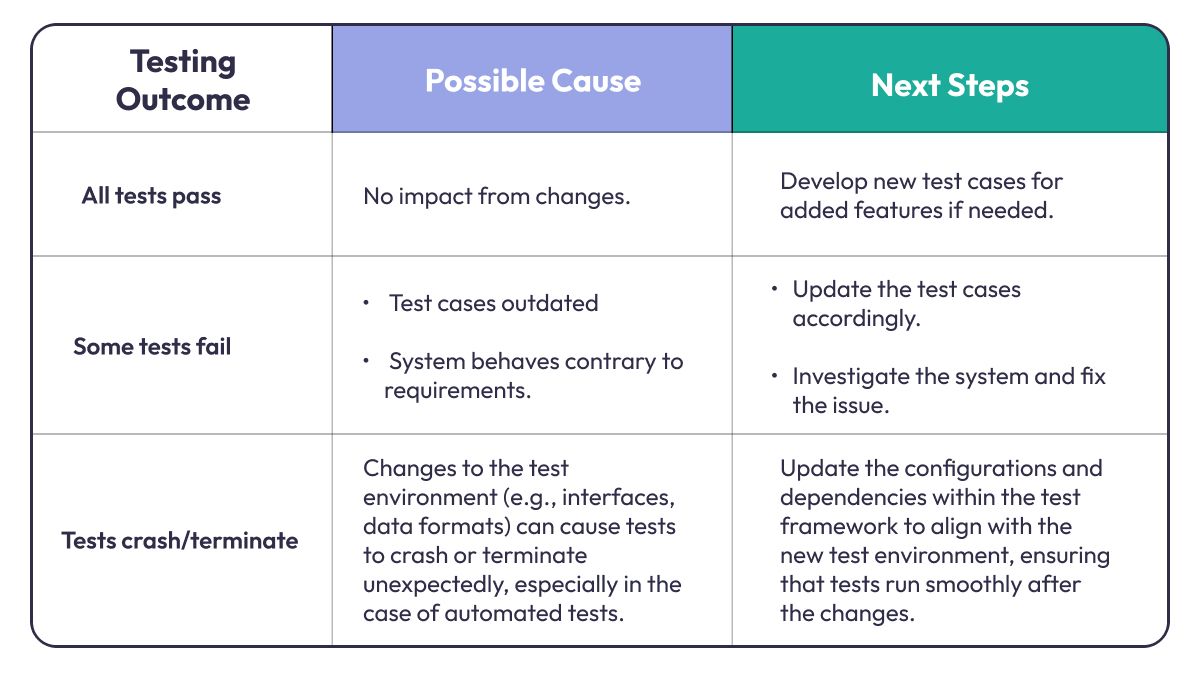
In cases where the test and system modules must be compiled into a single executable, changes to interfaces may prevent not only test execution but also compilation. In such scenarios, it's necessary to analyse the changes and update the test environment accordingly.
Sometimes, it's impossible to rerun the full test suite, either due to time constraints or the lengthy duration of all tests. In these situations, a common approach is selective testing, focusing only on parts of the system that are affected by changes. Full regression tests are performed only after a substantial accumulation of changes or at critical project milestones.
Stages of Regression Testing
The regression testing process typically includes:
- Analysis of changes made to the system (for selective testing)
- Selection of relevant test cases( for selective testing)
- Execution of test cases (test run)
- Analysis of test results
- Reporting of found defects
- Updating the test cases and dependencies (if needed)
Tips for Regression Testing
- Ensure the possibility of executing all test cases that verify the system’s functionality, or perform an analysis to determine which test cases should be rerun based on the changes.
- Develop test cases and environments using methods that facilitate easy updates when changes are made to the system.
- Design test cases in such a way that prevents residual data from one test from affecting the results of others.
The ultimate goal of regression testing is to continuously provide testers and developers with up-to-date and reliable information about the system's current state. Curious about testing in Agile? Don’t miss our complete guide on regression testing in Agile, when to do it, how to prioritise tests, and more.
When and Why Do You Need Both Testing Types?
Unit tests are your first line of defence. They're designed to validate the functionality of individual components—functions, methods, or classes—in isolation. These tests are typically fast, easy to automate, and should be triggered with every code change.
When You Need Both Types
- Developing new features
- Refactoring existing code
- Fixing bugs at the component level.
Unit tests are executed before the new or updated code is integrated into the system, typically by the developer to catch issues early within the component itself. Regression tests are performed after the code has been merged, ensuring that new changes haven’t unintentionally broken other parts of the system.
Why You Need Both Types
Running only unit tests gives you confidence in isolated components, but offers no guarantees about how they work together. On the other hand, relying solely on regression tests might uncover integration issues too late in the development cycle.
Using both ensures:
- Fast feedback loops (unit tests)
- System-wide reliability (regression tests)
- Early detection and lower cost of fixing bugs
- Smoother integration and deployment.
In short, unit testing secures your code’s foundation, while regression testing protects your product’s integrity over time. (Want top QA professionals without the hassle? Let’s talk about hiring QA engineers with us.)
Differences Between Regression Testing and Unit Testing
This table compares key differences of unit testing vs regression testing, helping you understand the distinct purposes and contexts in which each type of testing is used.
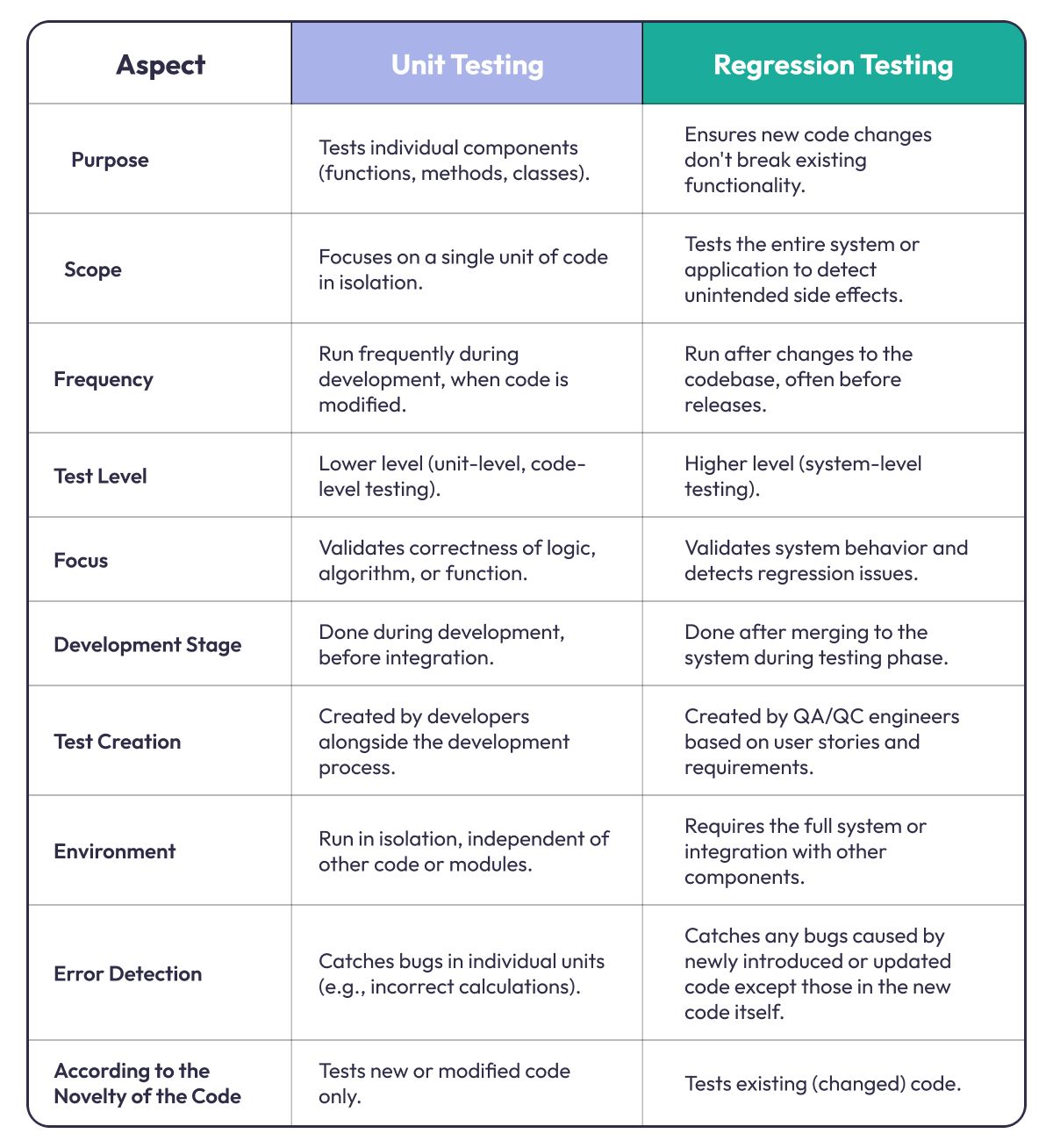
Unit and regression testing fulfil distinct functions throughout different phases of software development. Unit testing examines discrete components individually and occurs during the initial development stage. The purpose of regression testing is to examine the entire system for any disruptions to existing functionality caused by recent code updates. Both testing types work in tandem to guarantee that new code functions correctly and the entire application remains stable.
Conclusion
In conclusion, both unit testing and regression testing help ensure high-quality software but serve different purposes. Unit tests focus on individual components, ensuring each code unit works correctly in isolation. Regression tests, however, ensure that changes to the codebase do not negatively impact the overall system. Using both types of testing strategically, software teams can identify issues early and prevent defects from impacting the user experience. Let Luxe Quality do the heavy lifting for you. Tell us about your project, and we'll gladly help you.
Comments
There are no comments yet. Be the first one to share your opinion!
For 8 years, we have helped more than 200+ companies to create a really high-quality product for the needs of customers.
- Quick Start
- Free Trial
- Top-Notch Technologies
- Hire One - Get A Full Team
Was this article helpful to you?
Looking for reliable Software Testing company?
Let's make a quality product! Tell us about your project, and we will prepare an individual solution.
Unit testing verifies the proper functioning of software components, allowing developers to identify errors early in development and improve code quality.
No, regression testing cannot replace unit testing. While regression testing checks for issues in the overall system, unit testing is essential for testing the correctness of individual units or functions.
Regression testing should be conducted after all major codebase modifications, such as bug fixes and feature updates, before the final software release.
Unit tests examine system components separately, while regression tests assess the entire system for functionality disruptions that result from new changes.
The automation of regression testing becomes possible through various testing frameworks and tools that execute a predefined set of tests across the system for efficient and consistent testing results.